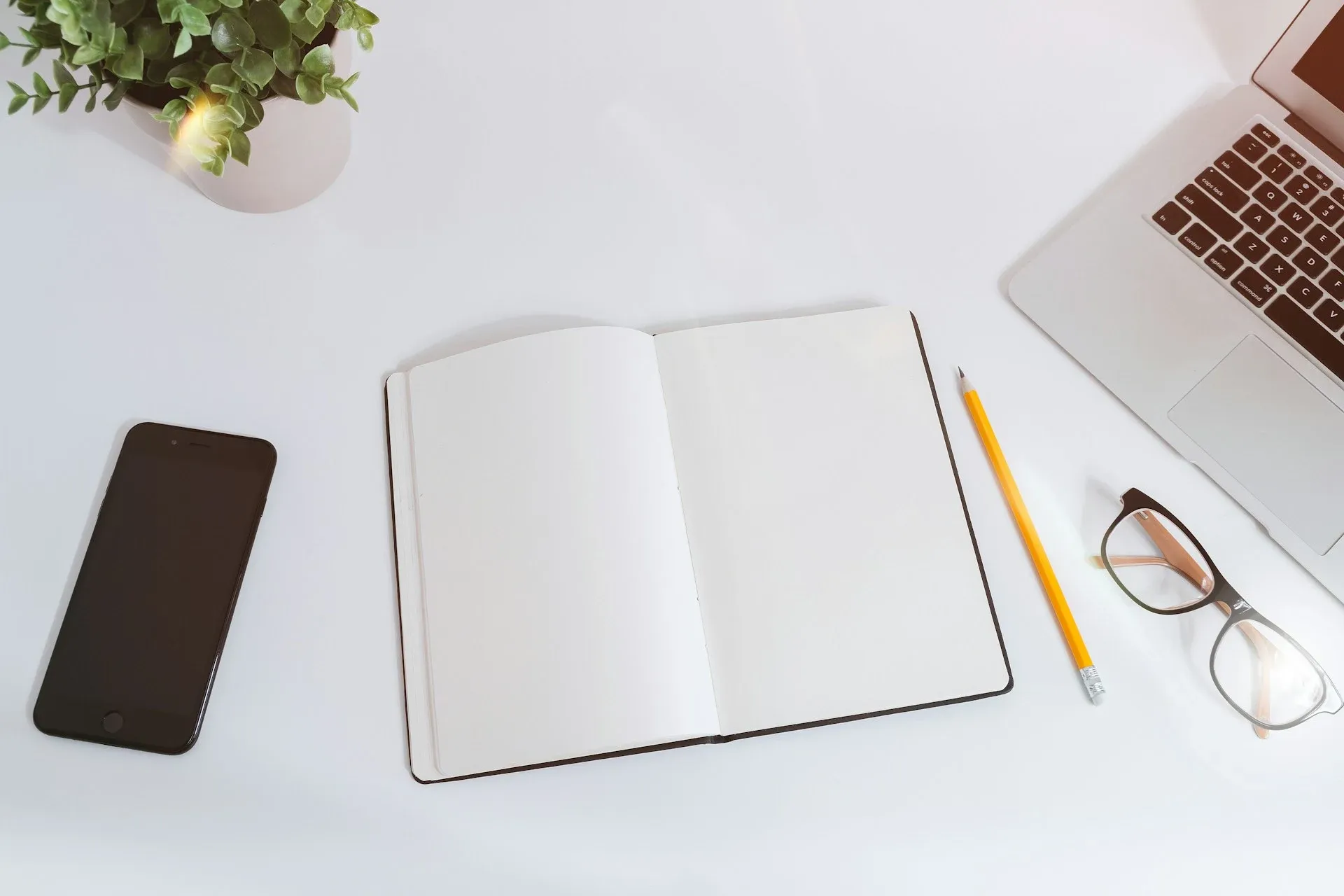
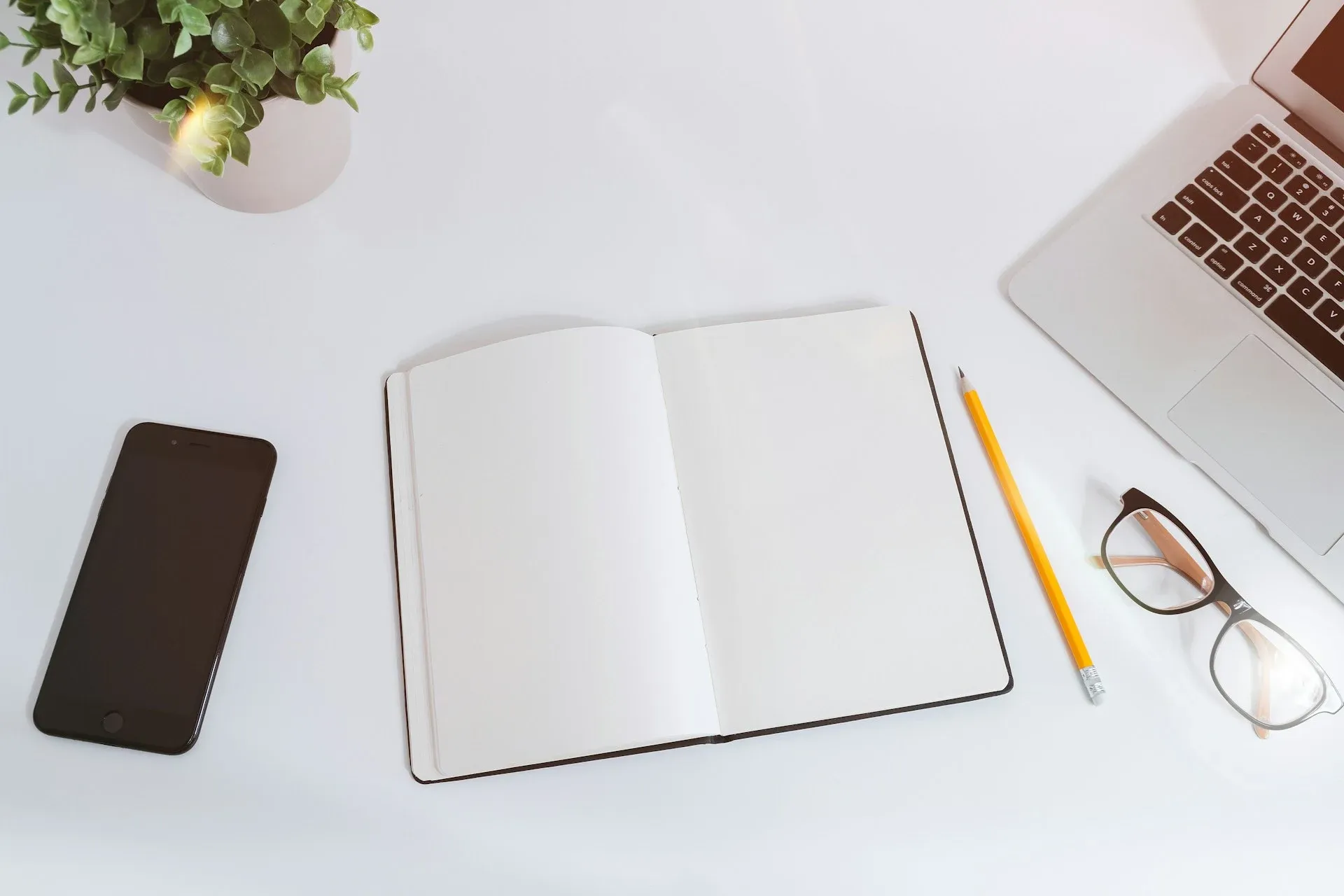
Master JavaScript for Your Next Big Interview
Comprehensive Content for learning Web Dev - From Basics to Advanced
Use this coupon 'PIYUSHAM19615' to get extra 10% discount on the course
Start LearningDocker Demystified: Understanding Image File And Docker Stages
Learn the basics of Docker Files. Explore the Docker File Code and discover how it build the image out of it.
Docker File
What is a Dockerfile?
A Dockerfile is essentially a text document with a specific set of instructions that can be run by Docker to create a Docker image. It defines the base image to pull, software to install, files to copy over into the image and commands to be run when the image is created.
How does a Dockerfile work?
The "docker build" command reads the instructions from the Dockerfile and builds a new image accordingly. Unfortunately, we do not have any use cases or examples of a Dockerfile with N number of layers since each instruction contributes to a separate image layer on its own, meaning that Docker is able to reuse the layers that have already been built.
To build your own images.
From node // hey docker please spin up a container which has the base image as Node
COPY index.js /home/app/script.js
CMD ["node", "/home/app/script.js"]
docker build -t mycustomimg .
// -t is to give tag which is mycustomimg and . is for directory means current directory.
docker run -it mycustomimg
// complete running docker file
From node
COPY index.js /home/app/script.js
CMD [ "node", "/home/app/script.js" ]
How does a Port Mapping happen?
const express = require("express");
const app = express();
const PORT = 3001;
app.get("/", (req, res) => {
return res.json({ message: "Hello from docker server" });
});
app.listen(PORT, () => console.log(`Server started at PORT:${PORT}`));
From node
COPY index.js /home/app/script.js
COPY package.json /home/app/package.json
WORKDIR /home/app/
RUN npm install
CMD [ "node", "script.js" ]
docker run -it -p 8000:3001 customimg
How To check inside the image?
docker run -it customimg bash
Build Stages
The primary purpose of build stages is to optimize the build process by reducing the size of the final image and separating the different components of the build.
The final build stage typically includes only the necessary files and dependencies required to run your application. By using build stages, you can significantly reduce the size of the final image since it only contains the essential components.
#syntax=docker/dockerfile:experimental
FROM node:14.4.0-stretch AS build
# Update package lists and install awscli
RUN apt update && \
apt install awscli -y
# Set working directory
WORKDIR /usr/src/app
# Copy the entire context (current directory) into the container's working directory
COPY . ./
# Remove unnecessary directories
RUN rm -rf /usr/src/app/node_modules
RUN rm -rf /usr/src/app/.next
RUN rm -rf /usr/src/app/dist
# Build the application using npm
RUN npm run build
# Remove unnecessary directories again
RUN rm -rf /usr/src/app/node_modules
RUN npm install --production
# Start a new build stage using a lightweight Node.js image
FROM node:14-alpine
WORKDIR /usr/src/app
# Copy necessary files from the build stage
COPY --from=build /usr/src/app/.next/ /usr/src/app/.next/
COPY --from=build /usr/src/app/public/ /usr/src/app/public/
COPY --from=build /usr/src/app/node_modules/ /usr/src/app/node_modules/
COPY --from=build /usr/src/app/package.json /usr/src/app/
COPY --from=build /usr/src/app/next.config.js /usr/src/app/
COPY --from=build /usr/src/app/.env /usr/src/app/
COPY --from=build /usr/src/app/newrelic.js /usr/src/app/
# Expose port 3000 for the application
EXPOSE 3000
# Start the application using the 'npm start' command
CMD npm start
Build Stage:
Uses the
node:14.4.0-stretch
base image.Installs the
awscli
package.Sets the working directory to
/usr/src/app
.Copies the entire context (current directory) into the container.
Removes unnecessary directories.
Builds the application using
npm run build
.Creates directories and copies specific files for deployment.
Removes unnecessary directories again.
Installs production dependencies.
Final Stage:
Uses the lightweight
node:14-alpine
base image.Sets the working directory to
/usr/src/app
.Copies necessary files from the build stage, including the
.next
directory, public files,node_modules
, configuration files, and environment files.Exposes port 3000.
Starts the application using
npm start
when the container is run
Read More
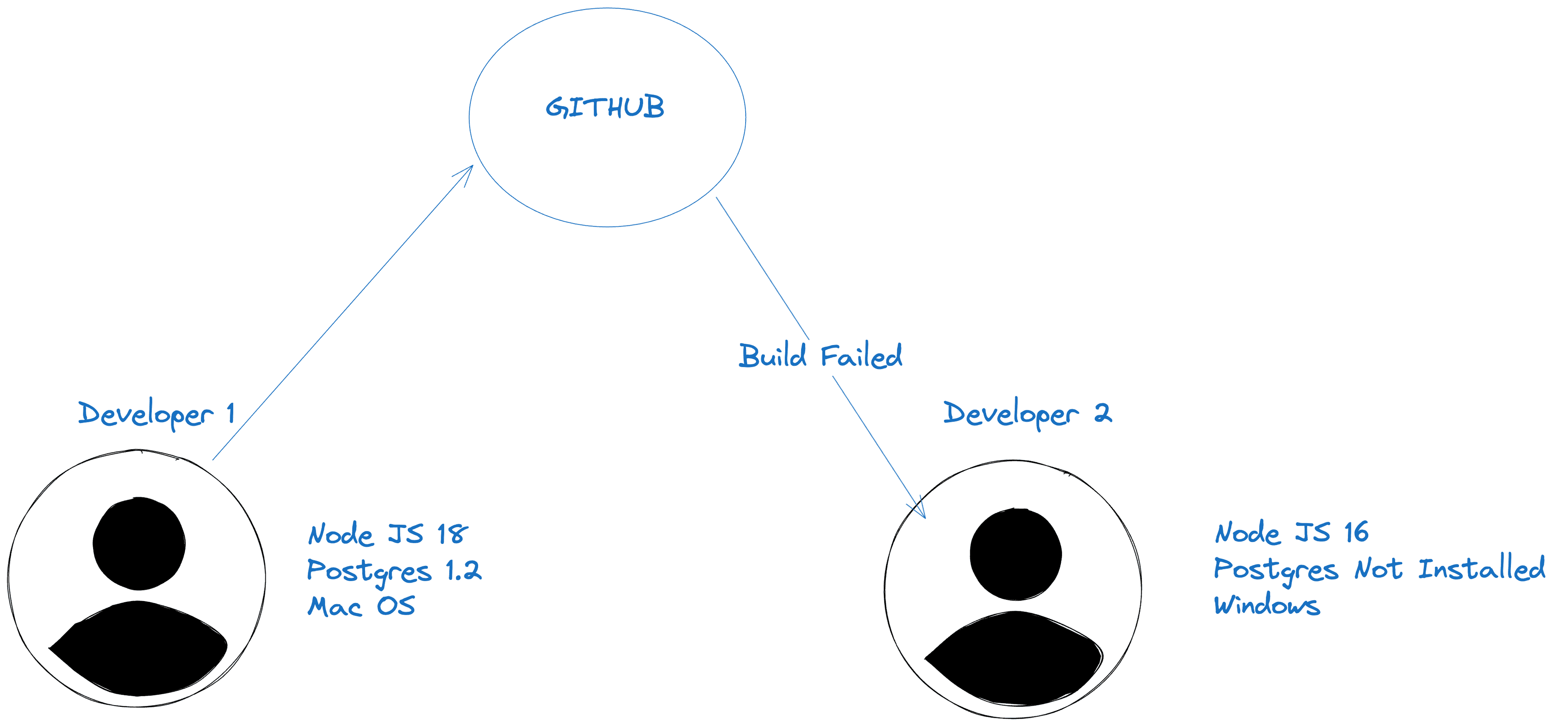
Docker Demystified: Understanding Containers, Images, and Architecture
Learn the basics of Docker containers and images. Explore the Docker architecture and discover how it simplifies application development and deployment.
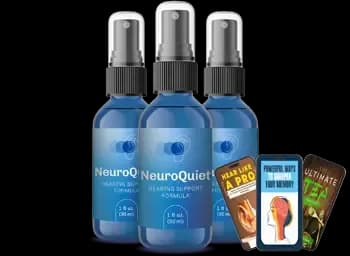
Boost Hearing & Focus with Neuro Quiet - Natural & Safe Supplement
Discover how Neuro Quiet enhances hearing health, detoxifies toxins, and improves cognitive clarity with its natural, safe ingredients. Guaranteed results or your money back
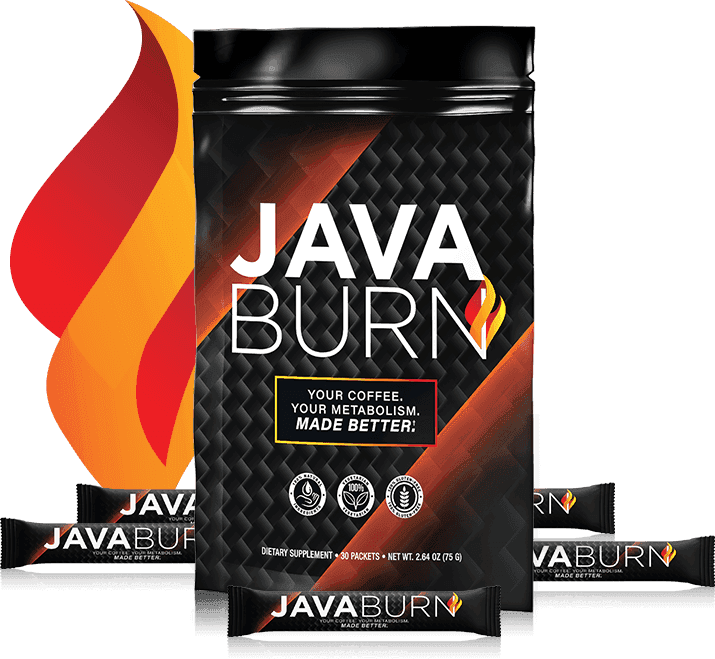
Java Burn: Natural Weight Loss Supplement with Holistic Benefits [55]
Explore the unique benefits of Java Burn, an all-natural supplement that boosts metabolism for long-term weight loss, enhances sleep quality, and reduces stress.
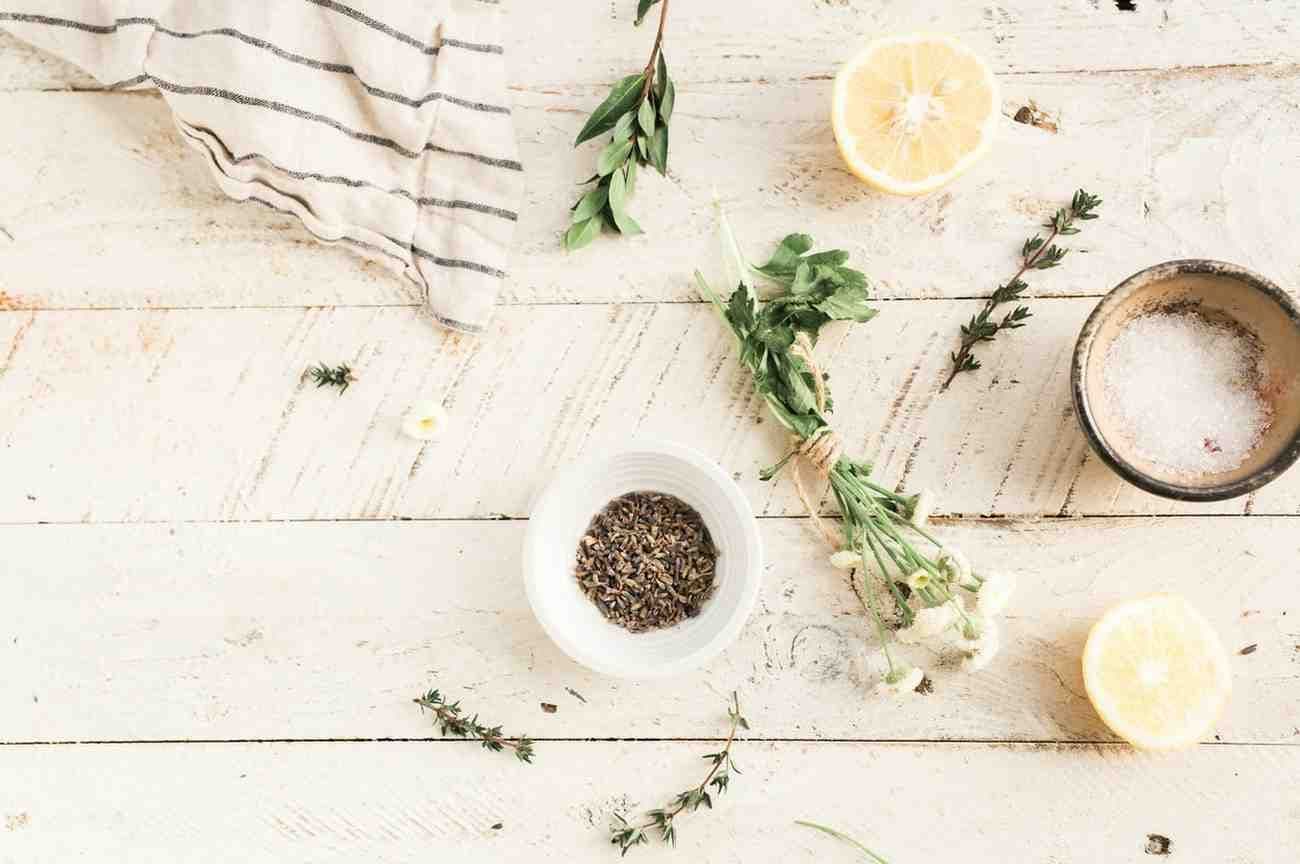
Niacinamide: Benefits and Uses in Skin Care
Learn how niacinamide, a multitasking vitamin B3, transforms your skincare routine by improving hydration, reducing discoloration, and fighting signs of aging.